How Builders Can Increase Their Debugging Abilities By Gustavo Woltmann
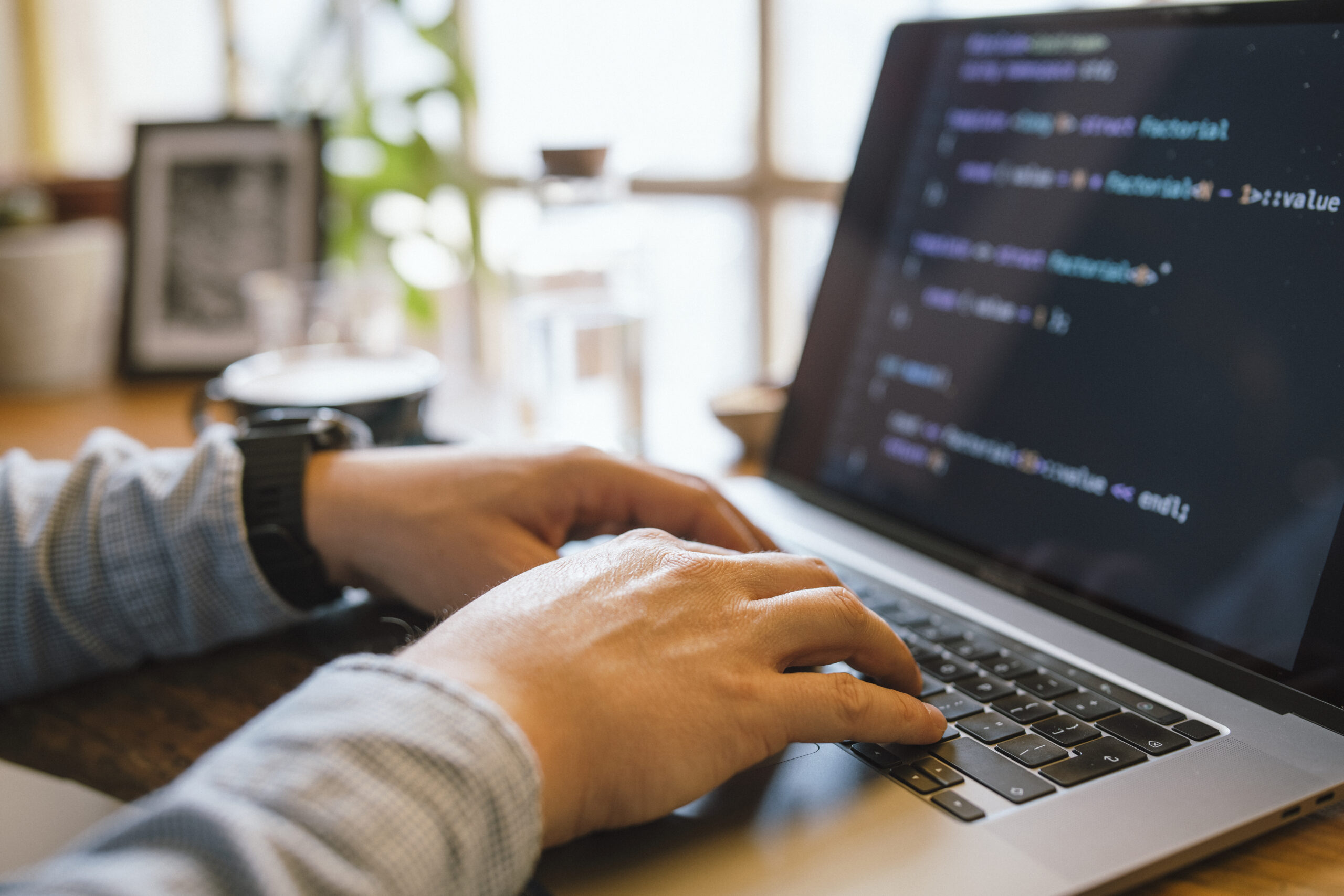
Debugging is One of the more important — nevertheless normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve difficulties proficiently. No matter if you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Here are quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is a single Portion of development, recognizing tips on how to communicate with it successfully during execution is Similarly crucial. Modern enhancement environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can do.
Take, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the value of variables at runtime, action via code line by line, and even modify code about the fly. When used effectively, they Allow you to notice precisely how your code behaves all through execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-finish developers. They assist you to inspect the DOM, check community requests, see true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch disheartening UI difficulties into manageable responsibilities.
For backend or program-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Regulate above functioning processes and memory administration. Learning these equipment can have a steeper Understanding curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be relaxed with version Management systems like Git to be aware of code history, locate the precise moment bugs had been launched, and isolate problematic variations.
Ultimately, mastering your resources signifies going past default settings and shortcuts — it’s about establishing an personal expertise in your development atmosphere to ensure when challenges arise, you’re not misplaced at midnight. The higher you already know your instruments, the greater time you could expend solving the particular trouble rather then fumbling as a result of the process.
Reproduce the Problem
Probably the most crucial — and often ignored — actions in efficient debugging is reproducing the problem. Prior to leaping in the code or building guesses, developers want to create a consistent environment or state of affairs wherever the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is accumulating as much context as possible. Check with inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The more detail you have got, the much easier it turns into to isolate the precise problems under which the bug happens.
When you’ve gathered sufficient info, seek to recreate the trouble in your neighborhood surroundings. This may imply inputting a similar knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions concerned. These checks not only support expose the problem but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it might take place only on selected functioning methods, browsers, or beneath individual configurations. Using equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But when you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes safely, and communicate much more clearly together with your team or buyers. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Go through and Realize the Error Messages
Error messages are often the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it occurred — if you know how to interpret them.
Start out by looking through the message diligently As well as in complete. Many builders, especially when less than time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it stage to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the responsible code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging method.
Some glitches are vague or generic, and in All those situations, it’s very important to examine the context through which the mistake occurred. Check out similar log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These normally precede larger concerns and provide hints about probable bugs.
Finally, error messages usually are not your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, supporting you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective instruments inside of a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, aiding you recognize what’s occurring underneath the hood while not having to pause execution or move in the code line by line.
A very good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for standard activities (like effective start-ups), Alert for probable troubles that don’t break the applying, Mistake for real problems, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your method. Deal with critical activities, state improvements, input/output values, and important determination points in your code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-assumed-out logging method, you may lessen the time it will take to identify click here challenges, acquire deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it is a type of investigation. To efficiently establish and fix bugs, developers need to technique the procedure similar to a detective resolving a mystery. This state of mind aids break down intricate difficulties into workable parts and adhere to clues logically to uncover the basis result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much related info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What might be triggering this habits? Have any adjustments lately been produced to the codebase? Has this difficulty happened ahead of below equivalent circumstances? The goal should be to slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue in a managed surroundings. If you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome guide you closer to the reality.
Shell out close awareness to tiny aspects. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and affected person, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and enable Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more practical at uncovering concealed problems in intricate devices.
Write Tests
Composing assessments is among the simplest ways to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just support capture bugs early but also serve as a safety net that provides you self confidence when building changes to your codebase. A effectively-examined application is simpler to debug as it helps you to pinpoint exactly where and when a problem occurs.
Start with unit tests, which concentrate on personal features or modules. These modest, isolated assessments can speedily reveal no matter if a certain bit of logic is Performing as envisioned. Any time a exam fails, you immediately know where to look, significantly reducing some time expended debugging. Device exams are Specifically helpful for catching regression bugs—issues that reappear after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclusion assessments into your workflow. These support make sure a variety of aspects of your software do the job with each other efficiently. They’re significantly handy for catching bugs that manifest in elaborate devices with numerous parts or expert services interacting. If a little something breaks, your assessments can inform you which Section of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to think critically regarding your code. To test a aspect appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from a discouraging guessing game into a structured and predictable approach—encouraging you catch a lot more bugs, speedier and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—observing your monitor for several hours, trying Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are far too near the code for too lengthy, cognitive fatigue sets in. You could commence overlooking clear problems or misreading code that you just wrote just hrs previously. In this particular condition, your brain turns into considerably less successful at dilemma-fixing. A short walk, a coffee crack, or simply switching to another undertaking for ten–quarter-hour can refresh your emphasis. Several developers report locating the root of a problem after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength along with a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well really feel counterintuitive, Primarily below limited deadlines, however it essentially leads to more rapidly and more effective debugging Over time.
To put it briefly, using breaks will not be an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your viewpoint, and allows you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Every single Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether or not it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Improper.
Start off by inquiring on your own some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught earlier with far better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or understanding and assist you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've uncovered from a bug with your friends might be Specifically powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team effectiveness and cultivates a more powerful Discovering lifestyle.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your improvement journey. In fact, many of the greatest builders will not be the ones who publish ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a fresh layer on your skill set. So upcoming time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.